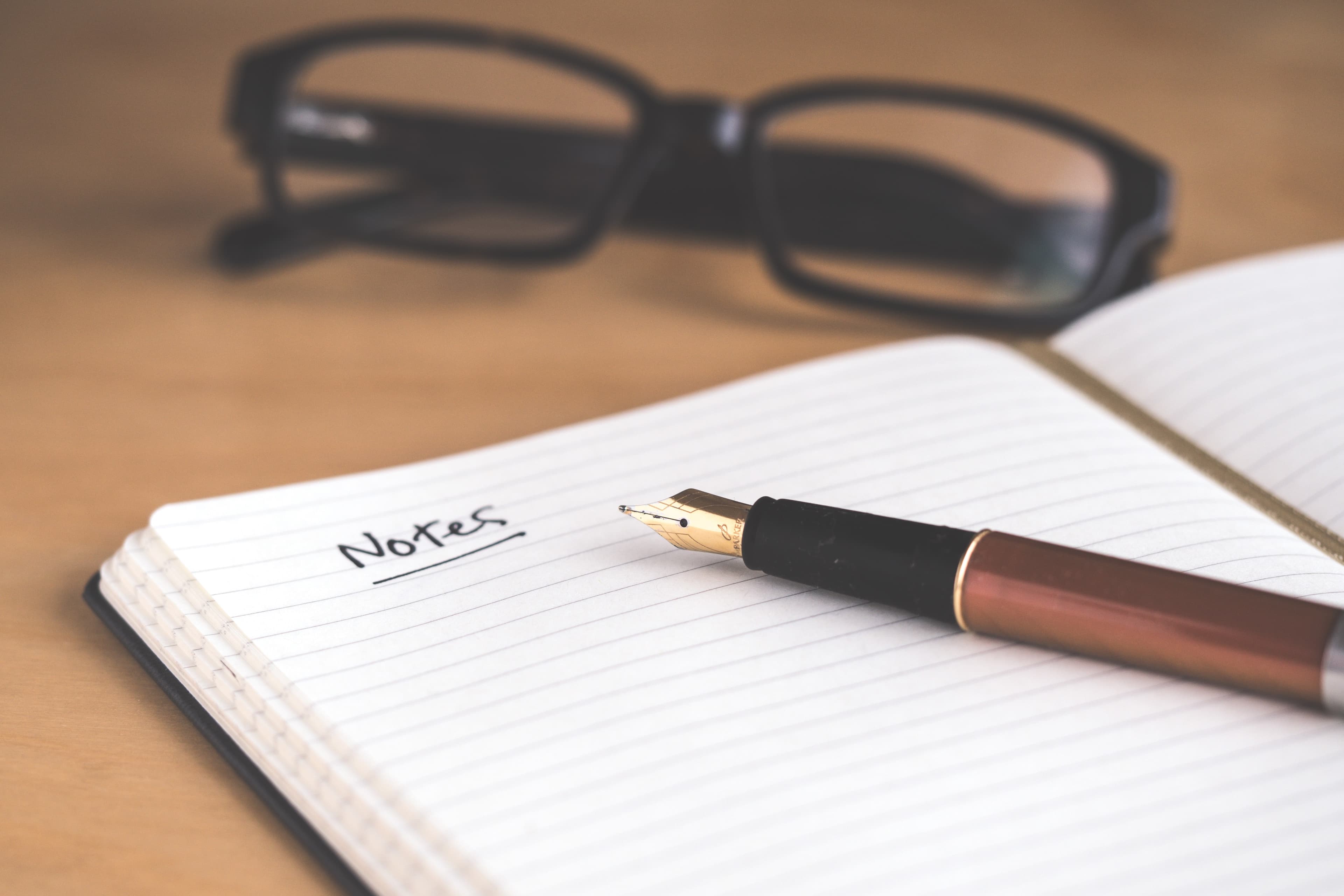
My CLI cheat-sheet
← Back to list
Last updated: June 2, 2020
Image by David Travis on Unsplash
***
There are some things that can be useful to me, but I totally keep forgetting the syntax. And I am tired of googling this each time.
Well, not any longer!
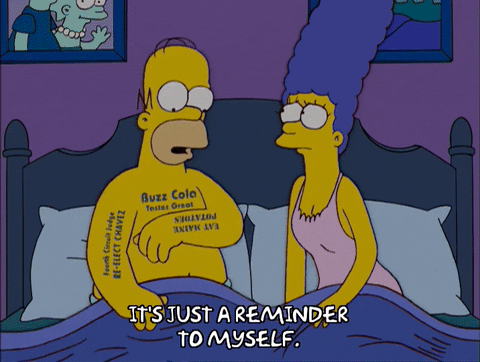
Image by simpsonsworld.com on Giphy
First, let me post my typical .bash_profile
file I use on every system:
👉 📃 ~/.bash_profile
export PS1='[\t]:\u@\W\$ 'export PATH=${PATH}:${HOME}/bin:${HOME}/.node/bin:${HOME}alias ll="ls -alFh"alias cp="cp -r"alias gitst="git status"alias gitar="git add -A"alias gitbr="git branch"alias gitcom="git commit -m"alias gitcomw="git commie -m wip"alias gituncom="git reset HEAD~1 --soft"alias gitchp="git cherry-pick -n"alias k="kubectl"alias kn="k -n <OUR_PROJECT>"alias restart_deployment='function __restartDeployment(){ kn scale deploy $1 --replicas=0; kn scale deploy $1 --replicas=$2; };__restartDeployment'alias docker_stop_all='docker stop $(docker ps -aq)'alias who_uses_port='function __whoUsesPort(){ lsof -n -i :"$1" | grep LISTEN; };__whoUsesPort'alias tfplan="terraform plan -out=/tmp/plan -no-color -detailed-exitcode"alias tfshowplan="terraform show -json /tmp/plan | jq '.resource_changes[] | select((.change.actions[] | contains(\"create\")) or (.change.actions[] | contains(\"delete\")) or (.change.actions[] | contains(\"update\"))) | (.change.actions | join(\",\")) + \": \" + .address'"tfplans() {terraform plan -no-color "$@" | grep -E 'be (replaced|destroyed|created)'}alias tfplans=tfplans
📃 Copy
# display branching treegit log --graph --full-history --all --pretty=format:"%h%x09%d%x20%s";git log --graph --decorate --oneline;# display history of commits for a specific branchgit log --walk-reflogs master;
📃 Copy
# remove last commit locallygit reset --hard HEAD~1# un-stage all uncommitted changesgit reset HEAD# un-stage all uncommitted changes and erase themgit reset HEAD --hard# remove un-tracked filesgit clean -fdgit clean -fX# get the amount of lines to be changedgit diff --cached --shortstat <BASE_BRANCH># reset unmerged changesgit reset file.txtgit checkout -- file.txt# abort the current unsuccessful mergegit merge --abort# revert without committinggit revert -n <COMMIT_HASH># cherry pick without committinggit cherry-pick -n <COMMIT_HASH># mergegit merge <BRANCH_NAME># rebaseget fetchgit rebase origin/mastergit push --force origin <BRANCH_NAME># show the last commit changesgit show --pretty=""
📃 Copy
# tag the current commitgit tag -a "tag_name"# push the taggit push origin "tag_name"# get all remote tagsgit fetch --all --tags# kill remote taggit push --delete origin v1.2.0# kill local taggit tag -d v1.2.0
📃 Copy
# add a submodulegit submodule add <REMOTE_URL> <DESTINATION_FOLDER># init (clone) all submodulesgit submodule update --init --recursive
📃 Copy
# do shallow clonegit clone <REPOSITORY> --depth 1# get has of the current commitgit rev-parse HEAD
📃 Copy
# parametrized aliasalias dssh='function __sampleAlias(){ docker exec -it $1 /bin/bash; };__sampleAlias'# get console command by its PIDps -p <PID> -o args# find where a binary is installedwhich <CLI_COMMAND># list all files sorting by date, human readable sizels -ltah# ... or reversels -ltahr# ... or by sizels -lSah# ... or by size reversels -lSahr# copy a file from a remote host 123.123.123.123 over ssh working on port 55332scp -P 55332 admin@123.123.123.123:/home/remote/path/to/file.gz ./# zip a folderzip -r folder.zip folder/# get file infofile ./file-name# find out PID of a process that opened a portlsof -n -i :<PORT> | grep LISTEN# show sub-folder sizes in the current folderdu -sh *# filter files by name against a wildcard, and deletefind . -name 'A*[0-9][0-9]' -delete# filter files by name against a wildcard, revert selection and deletefind . -not -name 'A*[0-9][0-9]' -delete# make group rights the same as the owner rightschmod -R g=u ./# redirect stdout and stderr to the same filiecmd > ./file.txt 2>&1# replace a string with another string in a filesed -e "s/one/another/g" -e "s/string1/string2/g" file.txt > altered_file.txt# list env varsprintenv# traverse jsondocker context inspect colima | jq -r '.[0].Endpoints.docker.Host'
📃 Copy
curl --request POST --header 'Authorization: token [TOKEN]' --url https://api.github.com/repos/[ACCOUNT]/[REPOSITORY_NAME]/actions/workflows/cd.yml/dispatches --data '{"ref":"master"}'
📃 Copy
# make a total package upgradeyarn upgrade <PACKAGE_NAME> --major# find out why the package was installedyarn why <PACKAGE_NAME># get the latest version of a package that exists on NPMyarn info <PACKAGE_NAME> | grep version:# install a package only for a current useryarn global add <PACKAGE_NAME> --prefix ~/.node# upgrade a chosen version of a packageyarn upgrade <PACKAGE_NAME>@^
📃 Copy
# build an imagedocker build -t [vendor_name]/[image_name]:[version] .# run an imagedocker run [vendor_name]/[image_name]:[version]# run an image in daemon modedocker run -d [vendor_name]/[image_name]:[version]# run an image of a web application interactively, and forward a portdocker run -it -p [host_port]:[image_port] [vendor_name]/[image_name]:[version]# push an image to dockerhubdocker login -u [username]docker push [vendor_name]/[image_name]:[version]# "ssh" into a container with ID=[container_id]docker exec -it [container_id] bash# see all running containersdocker ps# see logs of a container with ID=[container_id]docker logs [container_id]# copy file "config.txt" into a container with ID=[container_id] into /root folderdocker cp ./config.txt [container_id]:/root/# remove all exited containersdocker rm $(docker ps -a -f status=exited -q)# remove all working containersdocker rm $(docker ps -a -q)# remove all imagesdocker rmi $(docker images -a -q)# remove volumes, networksdocker system prune
📃 Copy
# run a command inside of a container without ssh into itdocker-compose exec [CONTAINER_NAME] [COMMANDS]
📃 Copy
# list podskubectl get pods# exec a command on a podkubectl exec -it [pod_name] [cmd]# get logs of a podkubectl logs [pod_name]# delete a podkubectl delete pod [pod_name]# apply a config filekubectl apply -f [config_file]# get information about a podkubectl describe pod [pod_name]# list deploymentskubectl get deployments# get information about a deploymentkubectl describe deployment [deployment_name]# delete a deploymentkubectl delete deployment [deployment_name]# apply rolling update on a deploymentkubectl rollout restart deployment [deployment_name]# list all namespaceskubectl get namespace# list services in a particular namespacekubectl get services -n [namespace]# forward a port from a pod to the hostkubectl port-forward [pod_name] [host_port]:[container_port]
📃 Copy
# dump a database (will create database_name/[collections])mongodump --db database_name# import a database (will import all collections in database_name/ folder)mongorestore -d database_name database_name# mongo shellmongosh# commands:use [DB_NAME] # use a databaseshow collections # list collectionsdb.[COLLECTION_NAME].find() # query elementsexit # quit
📃 Copy
# install psqlsudo apt install postgresql-client-common postgresql-client# connect to a databasepsql postgresql://[USERNAME]:[PASSWORD]@localhost/[DB_NAME]# commands:\l # list all databases\c [DB_NAME] # use a database\dt+ # list tables\q # quit psql# dump a databasepg_dump --user test --port=5432 --host=127.0.0.1 [DB_NAME] > dump.sql# create a new databasepsql --user=test --port=5432 --host=127.0.0.1 template1 -c 'create database "[DB_NAME]" with owner test'# import a databasepsql --user=test --port=5432 --host=127.0.0.1 [DB_NAME] < dump.sql
📃 Copy
# pull the remote state and save it to a fileterraform state pull > state.tfstate# push the remote state backterraform state push state.tfstate# to unlock the remote stateterraform force-unlock [LOCK-ID]# to get the names of the resources to be created or deletedterraform plan -out=plan -no-color -detailed-exitcodeterraform show -json plan | jq '.resource_changes[] | select((.change.actions[] | contains("create")) or (.change.actions[] | contains("delete"))) | (.change.actions | join(",")) + ": " + .address'# to plan for destructionterraform plan -out=plan -no-color -detailed-exitcode -destroy# fix formattingterraform fmt -recursive# show formatting issues without fixingterraform fmt -check -recursive —diff
📃 Copy
# launch chrome from the command line in Macalias chrome="/Applications/Google\\ \\Chrome.app/Contents/MacOS/Google\\ \\Chrome"# drop the "Cannot open an app from unidentified developer" restriction for a particular applicationsudo xattr -rd com.apple.quarantine /Applications/SomeApp.app# format a usb flash stickdiskutil list externaldiskutil eraseDisk MS-DOS "<label>" MBR <identifier># mount an iso image as an external drivehdiutil mount <path_to_image.iso>diskutil unmount /dev/<identifier>
📃 Copy
***
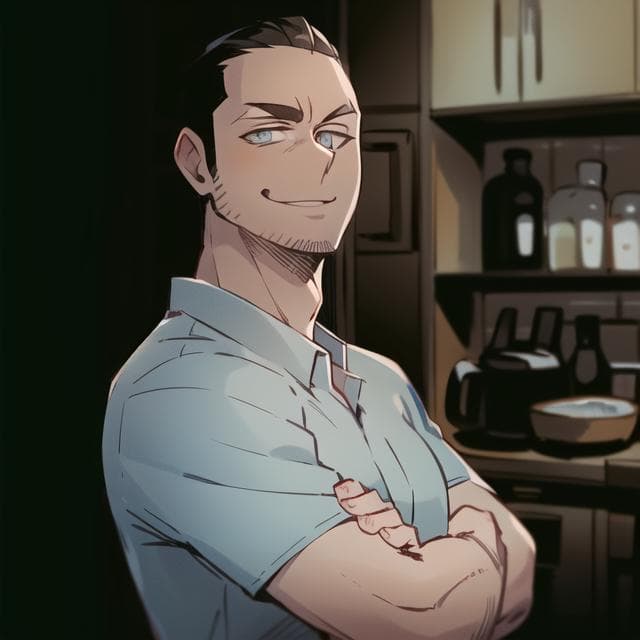
Sergei Gannochenko
Business-focused product engineer, in ❤️ with tech and making customers happy.
AI, Golang/Node, React, TypeScript, Docker/K8s, AWS/GCP, NextJS
20+ years in dev