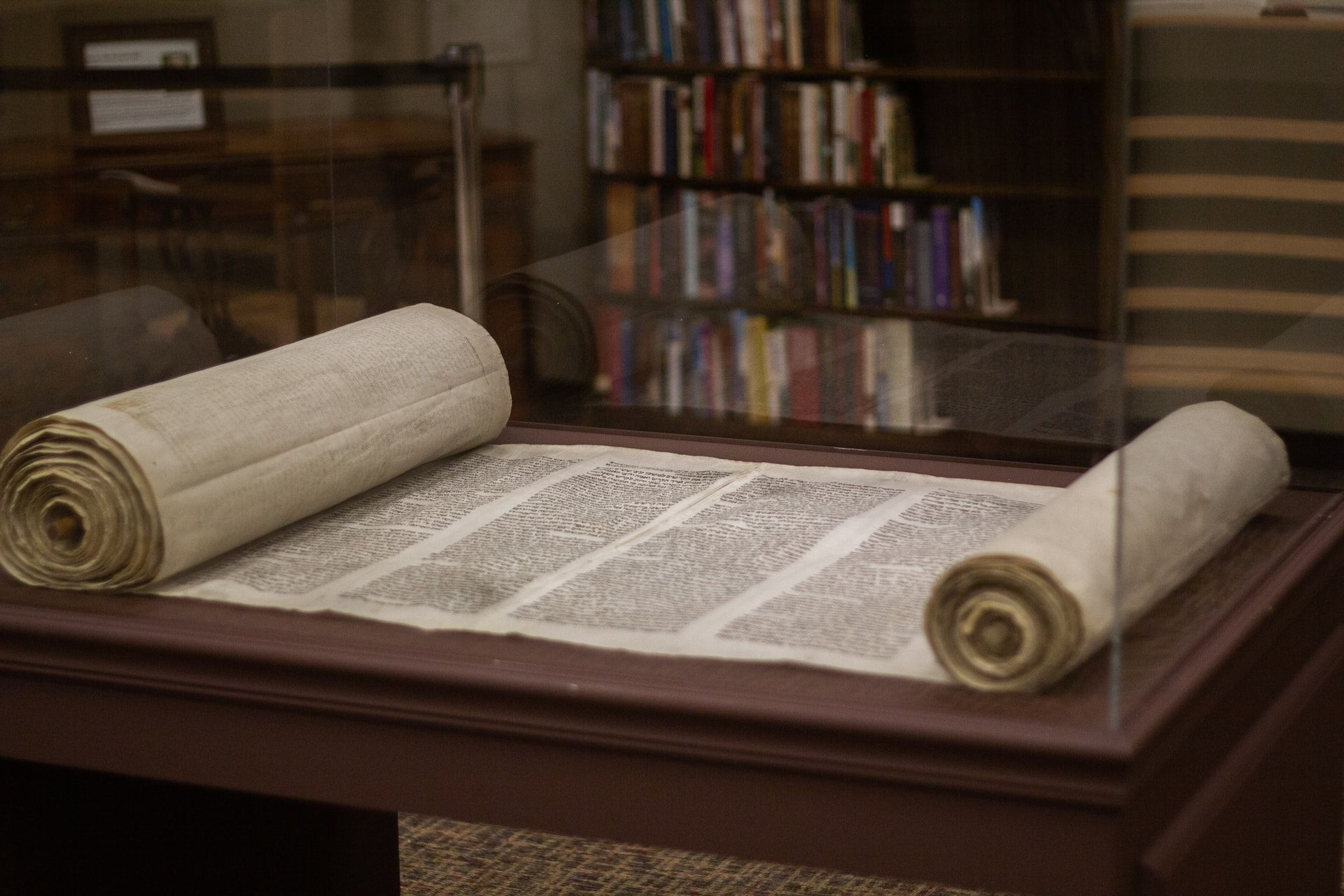
My Shell Script cheat-sheet
← Back to list
Posted on 03.06.2020
Last updated on 18.07.2021
Image by Taylor Wilcox on Unsplash
Refill!
Can't say I use shell scripting a lot, but when I do, I am always struggling with remembering certain useful syntax. So, this is just a reminder for the most frequently-used syntax.
Save command output to a variable
$
LIST=$(ls -l)
The code is licensed under the MIT license
Get the basename of the full path to the script file
$
DIR="$( cd "$( dirname "${BASH_SOURCE[0]}" )" && pwd )"
The code is licensed under the MIT license
Assign the default value to a variable
$
VERSION="${1:-latest}"
The code is licensed under the MIT license
Execute a command for each file in the folder
$
FOLDER="$(pwd)"for file in ${FOLDER}/*dols -l "$file"done
The code is licensed under the MIT license
Get basename of a path
$
basename /foo/bar/baz/wheel.txt
The code is licensed under the MIT license
Check if file exists
$
FILE=/etc/hostsif [[ -f "$FILE" ]]; thenecho "$FILE exists."elseecho "$FILE does not exist."fiif [[ ! -f "$FILE" ]]; thenecho "$FILE does not exist."elseecho "$FILE exists."fi
The code is licensed under the MIT license
Read environment variables from a file and define them
The variables will be only visible to the current process.
$
if [ -f .env ]thenexport $(cat .env | xargs)fi
The code is licensed under the MIT license
Default aliases and startup settings
On every new machine I prefer having some predefined aliased and settings I am used to. So normally I write this:
👉 📃 ~/.bash_profile
$
export PS1='[\t]:\u@\W\$ 'export PATH=${PATH}:${HOME}/binalias ll="ls -alFh"alias cp="cp -r"
The code is licensed under the MIT license
I always keep forgetting to apply it immediately afterwards ^^
$
source ~/.bash_profile
The code is licensed under the MIT license
Prompt a user
$
read -p "Running this script will sell your kidney. Proceed? " -n 1 -rechoif [[ $REPLY =~ ^[Yy]$ ]]then# dangerous operation herefi
The code is licensed under the MIT license
Create functions
$
function enterDir {echo "Entering $1"pushd $1 > /dev/null}enterDir /tmp/foo
The code is licensed under the MIT license
Set the script to stop on error
$
set -e
The code is licensed under the MIT license
Process arguments
$
while getopts a:b:c: flagdocase "${flag}" ina) OPTION_A=${OPTARG};;b) OPTION_B=${OPTARG};;c) OPTION_C=${OPTARG};;*) exit 1esacdoneecho ${OPTION_A}echo ${OPTION_B}echo ${OPTION_C}
The code is licensed under the MIT license
This can be really powerful when combined with the default value setter.
Check if a command is available
$
if ! command -v <command_name> &> /dev/nullthenecho "The command was not found"exitfi
The code is licensed under the MIT license
Get the exit status of the latest command
$
ls fooif [[ $? -ne 0 ]]; thenecho "No such directory"fi
The code is licensed under the MIT license
Replace strings in a file
Not exactly related to Shell scripting, but still could be useful in many cases.
$
@awk '{sub("fromstring","tostring")} {print}' ./.env.sample > temp.txt@mv temp.txt ./.env.test
The code is licensed under the MIT license
or, better:
$
sed -e "s/one/another/g" -e "s/string1/string2/g" file.txt > altered_file.txt
The code is licensed under the MIT license
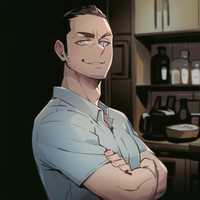
Sergei Gannochenko
Business-oriented fullstack engineer, in ❤️ with Tech.
Golang, React, TypeScript, Docker, AWS, Jamstack.
15+ years in dev.
Golang, React, TypeScript, Docker, AWS, Jamstack.
15+ years in dev.